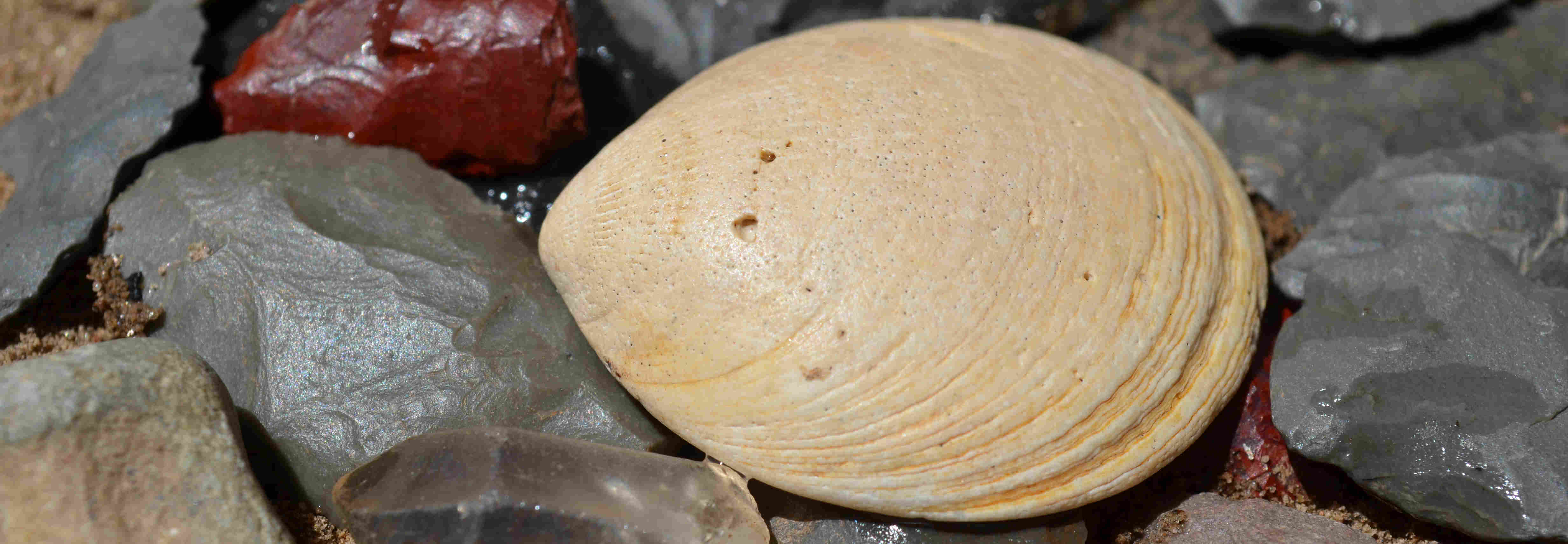
Overview
Bash is a tool that provides a text-based user interface for managing operating system resources. It’s also the run-time environment for a simple interpreted programming language that can be used to create scripts for automating tasks.
Bash is the evolution of the popular Born Shell (sh), a de facto standard among Unix-like operating systems. It provides all the features of its predecessor plus a new set of built-ins and configuration settings.
Using Bash
There are three common use cases for Bash:
- User shell: configured at the operating system level, provides the shell for user login, either local or remote.
- Script run-time: provides the execution run-time for Bash scripts
- Temporal shell: provides an interactive shell session on top of the user shell. The new session is a sub-process of the actual user or a different user when using privilege delegation tools such as SuDO.
This tutorial will focus on the scripts run-time use case.
Script structure
Bash scripts are text files describing a sequence of commands:
- Commands can be either built-in or external Linux apps.
- Commands are separated from each other by the new-line character (standard line separator for Linux/Unix systems).
- Long lines can be split using the scape character
\
- Commands can be grouped on the same line using the semicolon separator
;
- Commands and arguments are separated using one or more spaces.
- Although not required, it’s recommended that the first line of the script contains:
#!/bin/bash
- Script files must have execution and read permissions if used directly, or just read-only permissions if called using Bash.
For example:
|
|
Using Variables
Declaration
Variables in Bash are created using a declaration command and the equal =
symbol to associate the variable name to its value. Notice that there should be no spaces before and after the assignment symbol:
declare variable_name='variable value'
Bash provides the following built-in commands for declaring variables:
Command | Usage | Scope |
---|---|---|
export |
Declare global variables | Script-wide and sub-processes |
readonly |
Declare readonly variables (constants) | Script-wide |
declare |
Declare script level variables | Script-wide |
local |
Declare function level variables | Function-wide |
Consider the following best practices:
- Declare variables at the beginning of the code block.
- Declare and initialize all the variables that the script will use.
- Readonly and exported variables should be named using all uppercase.
- Use single quotes for static content.
Retreive variable’s value
To use the value of a variable, prepend the dollar symbol $
to the name of the variable surrounded by brackets {}
.
As a best-practice, surround variable expansion with double quotes:
echo "${test_variable}"
Bash also provides processing functions that can be used for altering the value before the expansion.
For example:
|
|
Data Types
Bash supports four data types:
Type | Declaration command |
---|---|
string | declare |
integer | declare -i |
array | declare -a |
associative array | declare -A |
Even though there is no explicit boolean data type, Bash interprets the exit status of commands as:
- exit status == 0: true
- exit status > 0: false
Parameters
Parameters are special variables that are automatically created when a function or script is called with arguments.
The variable name is created by using a single number to represent the position of the word in the argument list:
bash say_hello.bash 'to' 'my' 'little' 'friend'
|
|
Shell Expansion
In addition to variables Bash provides additional features for generating dynamic values:
$( )
: Command Expansion: Execute the command in a sub-process and expand its STDOUT.$(( ))
: Arithmetic Expansion: Evaluate the arithmetic expression and use the resulting value for the expansion.$(< )
: File Expansion: Read the content of a file and use it for the expansion.
For example:
Working with processes
Before going into the details, let’s review the following key concepts about Unix processes:
- A process is a running app that is executed from an already running process (parent). For scripts, the parent process is the one running the Bash run-time.
- The Operating System assigns unique integer identifiers to each process (PID: Process ID).
- Processes have 3 data paths:
- STDIN: standard input: process can read data from this path.
- STDOUT: standard output: process can write data to this path.
- STDERR:: standard error: process can write error diagnostic data to this path.
- Exit status: numeric value that represents the final execution status of the process. In general:
0
: successful execution>0
: failed execution. The app can assign different numbers to further describe the error.
Bash provides the following features for interacting with processes:
- Variables: show process information
$?
: exit status of the last executed command$BASHPID
: PID of the current Bash process
- Redirection: redirect the data flow from the STDIN, STDOUT, and STDERR
- Pipelines: integrate two processes by creating a pipe between the STDOUT from one to the STDIN of the other
Additional features are available but no covered in the current tutorial (jobs, signals, traps, parallelism, etc.)
Implementing Functions
To declare a function in Bash use the following structure:
Functions in Bash behave in a similar way to scripts and commands:
- Can be called directly from the script or command line
- Use positional parameters that are automatically assigned to
$N
variables - Have exit status
- Can send data to STDOUT and STDERR
- Can receive data from STDIN
- Can be used in complex command sequences (
&&
,||
,|
)
Let’s create a function with all the concepts seen so far:
|
|
Next Steps
Continue reading the second part of the tutorial: Bash Scripting Concepts: Part 2 of 2
Copyright information
This article is licensed under a Creative Commons Attribution 4.0 International License. For copyright information on the product or products mentioned inhere refer to their respective owner.
Disclaimer
Opinions presented in this article are personal and belong solely to me, and do not represent people or organizations associated with me in a professional or personal way. All the information on this site is provided “as is” with no guarantee of completeness, accuracy or the results obtained from the use of this information.