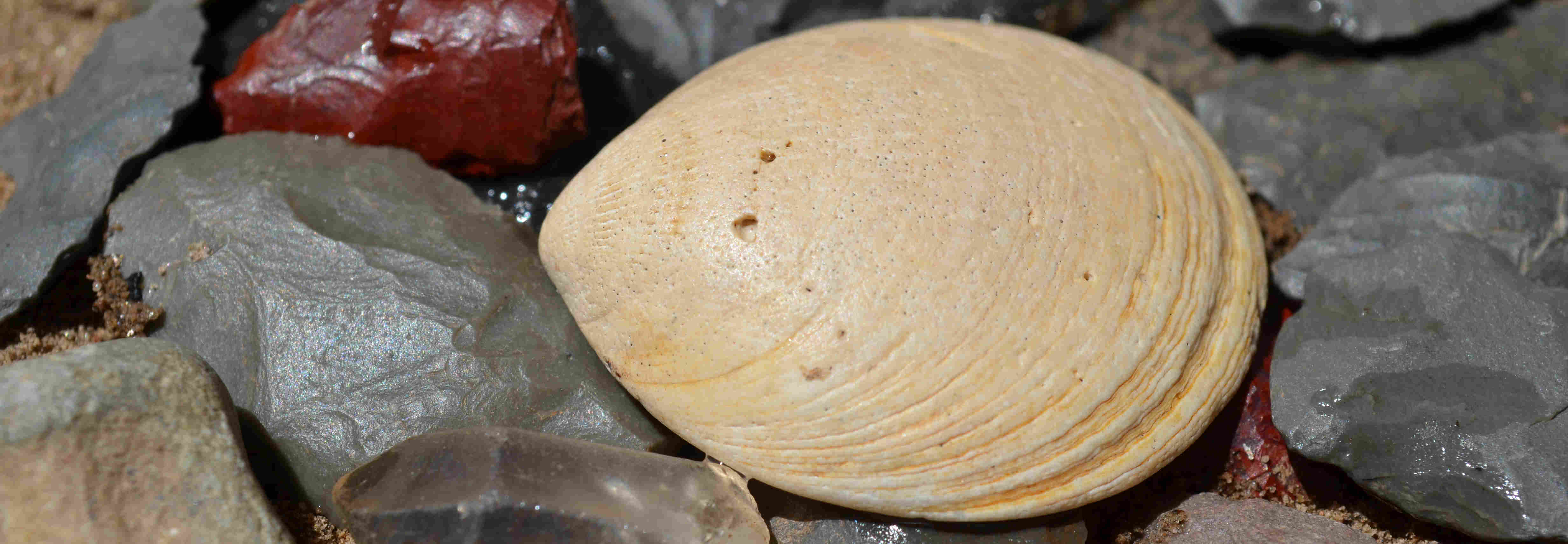
Overview
This is the second part of the tutorial. If not done already, please read the first part: Bash Scripting Concepts: Part 1 of 2
Working with loops
Bash provides three ways of creating loops:
for
: loop for a predefined number of timeswhile
: loop while the exit condition is true. The condition is evaluated before executing tasks.until
: loop until the exit condition is true. The condition is evaluated after executing tasks.
In addition to the loop condition, Bash provides two statements that can be used to control the loop execution flow:
break
: forces the loop to stop.continue
: forces the loop to skip remaining tasks and start the next iteration.
Command: while
In the following example, the loop iterates 5 times. The loop condition is evaluated after all commands in the code block are executed:
Command: until
Using the same structure as in the while
example, notice that now the loop iterates 4 times only. This is because the loop condition is evaluated before executing the code block:
Command: for
In the case of the for
loop the iteration is predefined. Instead of having a loop condition, the loop variable count
will be assigned each value in the list:
Working with conditionals
Bash provides the following options for implementing conditional execution:
||
: (logical OR) evaluates the execution of two commands and sets the exit status to zero if any associated exit status is zero.&&
: (logical AND) evaluates the execution of two commands and sets the exit status to zero if all associated exit statuses are zero.\!
: (logical NOT) evaluates the execution of a command and sets the exit status to zero if the associated exit status is not zero.(( ))
: performs logical evaluation on the integer expression and sets the exit status to zero if true[[ ]]
: evaluates the literal expression and sets the exit status to zero if true.if
: executes a command and if the exit status is zero then performs additional actions.case
: compares the provided value against a list of patterns and executes the commands upon match.
As mentioned before, Bash interprets the exit status of commands as:
0
: true>0
: false
In the following examples true
and false
are external commands that emulates true and false values (exist status 0 and 1 respectively)
Logical OR: ||
|
|
Logical AND: &&
|
|
Logical NOT: !
Arithmetic Expression Evaluation: (( ))
The (( ))
form accepts several logical operators. Some of them are:
==
: equal!=
: not equal>
: greater than<
: less than>=
: greater than or equal<=
: less than or equal
Expression Evaluation: [[ ]]
The [[ ]]
form accepts several logical operators and tests. Some of them are:
==
: equal!=
: not equal-z
: string is empty-n
: string is not empty-f
: path is a file
For ==
and !=
the special character *
can be used as a wildcard to match zero or more characters to the right.
Command: if
In the following example, arithmetic evaluation is used. Notice that quotes are not required within (( ))
|
|
Command: case
Redirecting data flows
Bash provides two alternatives for establishing data flows:
- Redirection
- Set read source for STDIN
- Set write destination for STDOUT
- Set write destination for STDERR
- Pipelines: integrate two commands by plugging the STDOUT from the first one to the STDIN of the second one
Redirection
In the following example, two functions will communicate with each other using a common file:
|
|
Pipelines
This example shows an alternative way of integrating both functions using pipelines:
Next Steps
Discover advanced features by exploring the Bash Reference Manual:
- jobs
- signals
- traps
- parallelism
- error handling
- configuration settings
Organize your code by choosing a coding style. For example: Google Shell Style Guide
Enhance script’s quality by incorporating linter and testing tools:
Copyright information
This article is licensed under a Creative Commons Attribution 4.0 International License. For copyright information on the product or products mentioned inhere refer to their respective owner.
Disclaimer
Opinions presented in this article are personal and belong solely to me, and do not represent people or organizations associated with me in a professional or personal way. All the information on this site is provided “as is” with no guarantee of completeness, accuracy or the results obtained from the use of this information.